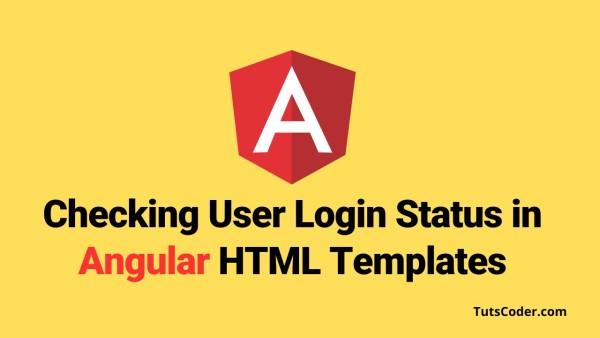
Checking User Login Status in Angular HTML Templates
It's common practice to design online applications with features or areas of the application that should only be accessible to logged-in users. In Angular, you may verify whether a user is signed in within an HTML template and then utilise a service to manage user authentication.
Let's begin by developing an authentication service with a mechanism to verify whether a user is currently logged in. In this example, we'll build a simple AuthService with an isLoggedIn() method that determines whether a user is logged in or not and returns a boolean answer.
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class AuthService {
isLoggedIn(): boolean {
// Replace this with your own authentication logic
return localStorage.getItem('currentUser') !== null;
}
}
This AuthService
simply checks if there is a currentUser
item in the browser's localStorage and returns true
if there is. You should replace this with your own authentication logic that is appropriate for your application.
Now that we have an authentication service, we can use it in an Angular component's HTML template to conditionally display content based on whether a user is logged in or not.
import { Component } from '@angular/core';
import { AuthService } from './auth.service';
@Component({
selector: 'app-category',
templateUrl: './category.component.html',
styleUrls: ['./category.component.css']
})
export class CategoryComponent {
constructor(private authService: AuthService) {}
isLoggedIn(): boolean {
return this.authService.isLoggedIn();
}
}
In this updated version of the CategoryComponent
, we still inject the AuthService
into the constructor, but instead of accessing the authService
directly, we create a public method called isLoggedIn()
that calls the isLoggedIn()
method of the authService
. This approach provides a layer of abstraction between the component and the authService
, making our code more modular and easier to maintain.
Now, in the category.component.html
template, we can use the isLoggedIn()
method to conditionally display content based on whether the user is logged in or not:
<div *ngIf="isLoggedIn()">
<!-- Content for logged-in users -->
<p>Welcome, {{ username }}!</p>
<button (click)="logout()">Log Out</button>
</div>
<div *ngIf="!isLoggedIn()">
<!-- Content for non-logged-in users -->
<p>Please log in to access this feature.</p>
<button routerLink="/login">Log In</button>
</div>
In this HTML template, we use Angular's built-in *ngIf
directive to conditionally display content based on the result of the isLoggedIn()
method. If the user is logged in, the first div
will be displayed with a welcome message and a log out button. If the user is not logged in, the second div
will be displayed with a prompt to log in and a button that links to the login page.
By creating a public method in the component that calls the private method of the service, we have created a more modular and maintainable solution that separates concerns and promotes code reuse.