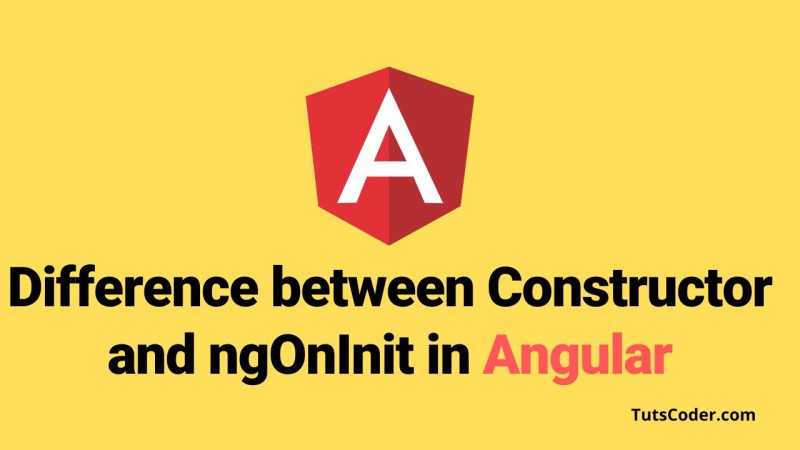
Understanding the Difference between Constructor and ngOnInit in Angular 15
In this article, we will see the difference between Constructor and ngOnInit in Angular.
When working with Angular, you may have come across two important functions - the constructor and ngOnInit. While both of these functions play a crucial role in an Angular application, they are used for different purposes. In this blog post, we will discuss the difference between constructor and ngOnInit in Angular 15, and when to use each.
Constructor in Angular
The constructor function in Angular is a special function that is executed whenever a new instance of a component is created. This function is called only once during the lifecycle of a component, and it is used for initializing the properties of the component.
The Constructor is not actually part of Angular, it is a feature of TypeScript class, which is called when the class is instantiated.
Constructor is used to create a new instance of the class.
The constructor will be automatically invoked by the JavaSscprt engine.
In Angular, the constructor is used for injecting dependencies into the component class.
While writing the code try to keep the constructor as simple as possible, so Unit Testing can be very easy if the constructor logic is simple.
Here is an example of a constructor function in Angular:
export class AppComponent {
title = 'My Angular App';
constructor() {
console.log('constructor');
}
}
NgOnInit
The ngOnInit function in Angular is another important function that is called after the constructor function. This function is part of the Angular component lifecycle and is called every time the component is initialized. It is used for initializing the data that the component needs to display.
Every component we create has a life cycle managed by Angular.
The NgOnInt is a life cycle hook managed by Angular.
Whenever you create a new component using angular-CLI ngOnint is added by default.
NgOnint will be called after the constrcor execution and after first NgOnchanges
NgOnInit() is called by Angular to indicate that Angular is done with initializing the component.
The NgonInit is called after the constructor is executed. In Constructor Angular initializes and resolve all class members so in NgonInit you can initialize work and logic the component.
Here is an example of an ngOnInit function in Angular:
export class AppComponent implements OnInit {
title = 'My Angular App';
constructor() {
console.log('constructor');
}
ngOnInit() {
console.log('ngOnInit');
}
}
Difference between Constructor and ngOnInit
The main difference between the constructor and ngOnInit functions in Angular is that the constructor function is called only once during the initialization of a component, while the ngOnInit function is called every time the component is initialized.
The constructor function is used for initializing the properties of a component, while the ngOnInit function is used for initializing the data that the component needs to display.
When to use Constructor and ngOnInit
Use the constructor function to initialize the properties of a component. This function is called only once during the lifecycle of a component.
Use the ngOnInit function to initialize the data that the component needs to display. This function is called every time the component is initialized.
Conclusion
Both the constructor and ngOnInit functions are important in Angular, and they are used for different purposes.
The constructor function is used for initializing the properties of a component, while the ngOnInit function is used for initializing the data that the component needs to display. Understanding the difference between these two functions is essential for building robust and efficient Angular applications.