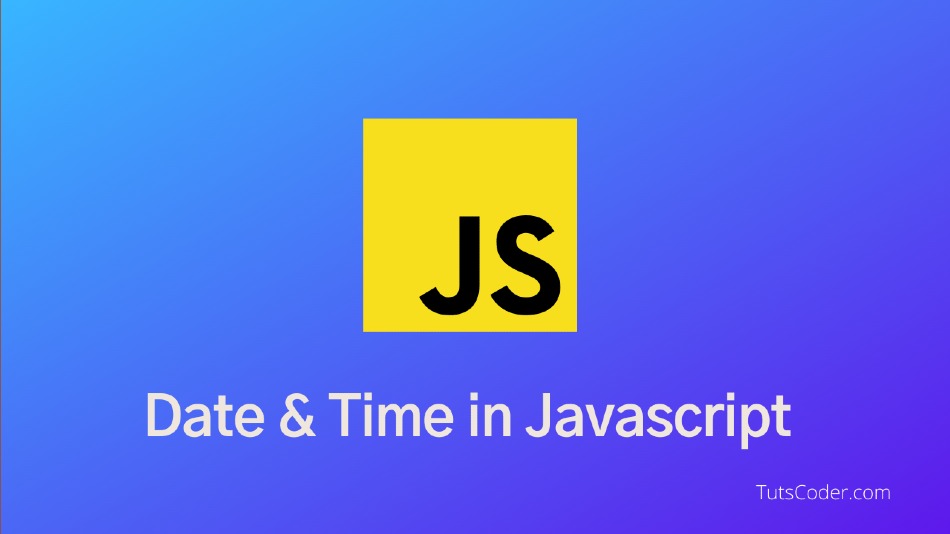
Date and Time in JavaScript Made Simple: Everything You Need to Know
In this tutorial, we will learn how to use the Date object in JavaScript with the help of examples.
What is the Date and Time in JavaScript?
JavaScript stores dates as a number of milliseconds since January 01,1970,00:00:00 UTC (Universal Time Coordinated).
In JavaScript, we only care about two—Local Time and Coordinated Universal Time (UTC).
- Local time refers to the timezone your computer is in.
- UTC is synonymous with Greenwich Mean Time (GMT) in practice.
By default, almost every date method in JavaScript (except one) gives you a date/time in local time. You only get UTC if you specify UTC.
What is a Date Object in JavaScript?
The Date object is a built-in javascript object, which allows you to get the user's local time by accessing the computer system clock through the browser.
Dates in JavaScript always reflect UTC time. Chrome browser displays the date in your local time zone by utilizing the time zone settings on your machine.
It also provides the date and time details and also supports various methods.
Creating a date
You can create a date with new Date(). There are four possible ways to use new Date():
- With no arguments
- With a date-string
- With date arguments
- With a timestamp
With no arguments
If you create a date without any arguments, you get a date set to the current time (in Local Time).
const currentDate = new Date();
console.log(currentDate);
//Output
Tue Oct 25 2022 17:16:48 GMT+0530 (India Standard Time)
In the above example. the new Date() will create a new date object with the current date and local time.
The date-string method
In the date-string method, you create a date by passing a date-string into new Date.
const currentDate = new Date('2022-10-25');
console.log(currentDate);
//Output
Tue Oct 25 2022 05:30:00 GMT+0530 (India Standard Time)
In JavaScript, if you want to use a date string, you need to use a format that’s accepted worldwide. One of these formats is the ISO 8601 Extended format.
`YYYY-MM-DDTHH:mm:ss.sssZ`
If Z is present, the date will be set to UTC. If Z is not present, it’ll be Local Time. (This only applies if time is provided.)
There’s a huge problem with creating dates with date strings.
If you live in an area that’s behind GMT, you’ll get a date that says 10th June.
If you live in an area that’s ahead of GMT, you’ll get a date that says 11th June.
If you create a date (without specifying time), you get a date set in UTC.
In the above scenario, when you write new Date('2019-06-11'), you actually create a date that says 11th June, 2019, 12am UTC. This is why people who live in areas behind GMT get a 10th June instead of 11th June.
If you want to create a date in Local Time with the date-string method, you need to include the time. When you include time, you need to write the HH and mm at a minimum (or Google Chrome returns an invalid date)
new Date('2019-06-11T00:00')
The whole Local Time vs. UTC thing with date-strings can be a possible source of error that’s hard to catch. So, I recommend you don’t create dates with date strings.
(By the way, MDN warns against the date-string approach since browsers may parse date strings differently).
If you want to create dates, use arguments or timestamps.
Creating dates with arguments
- Year: 4-digit year.
- Month: Month of the year (0-11). Month is zero-indexed. Defaults to 0 if omitted.
- Day: Day of the month (1-31). Defaults to 1 if omitted.
- Hour: Hour of the day (0-23). Defaults to 0 if omitted.
- Minutes: Minutes (0-59). Defaults to 0 if omitted.
- Seconds: Seconds (0-59). Defaults to 0 if omitted.
- Milliseconds: Milliseconds (0-999). Defaults to 0 if omitted.
// 11th June 2019, 5:23:59am, Local Time
new Date(2019, 5, 11, 5, 23, 59)
Many developers (myself included) avoid the the arguments approach because it looks complicated. But it’s actually quite simple.
The most problematic part with Date is that the Month value is zero-indexed, as in, January === 0, February === 1, March === 2 and so on.
Notice dates created with arguments are all in Local Time?
If you ever need UTC, you create a date in UTC this way:
// 11th June 2019, 12am, UTC.
new Date(Date.UTC(2019, 5, 11))
Creating dates with timestamps
In JavaScript, a timestamp is the number of milliseconds elapsed since 1 January 1970 (1 January 1970 is also known as Unix epoch time).
We mostly use this method to compare different dates.
const currentDate = new Date(1666698741392);
console.log(currentDate);
//Output
Tue Oct 25 2022 17:22:21 GMT+0530 (India Standard Time)
Formatting a date
The native Date object comes with seven formatting methods. Each of these seven methods give you a specific value (and they’re quite useless).
- toString gives you Wed Jan 23 2019 17:23:42 GMT+0800 (Singapore Standard Time)
- toDateString gives you Wed Jan 23 2019
- toLocaleString gives you 23/01/2019, 17:23:42
- toLocaleDateString gives you 23/01/2019
- toGMTString gives you Wed, 23 Jan 2019 09:23:42 GMT
- toUTCString gives you Wed, 23 Jan 2019 09:23:42 GMT
- toISOString gives you 2019-01-23T09:23:42.079Z
Conclusion:
Do let me know If you face any difficulties please feel free to comment below we love to help you.
if you have any feedback suggestion then please inform us by commenting.
Don’t forget to share this tutorial with your friends on Facebook and Twitter