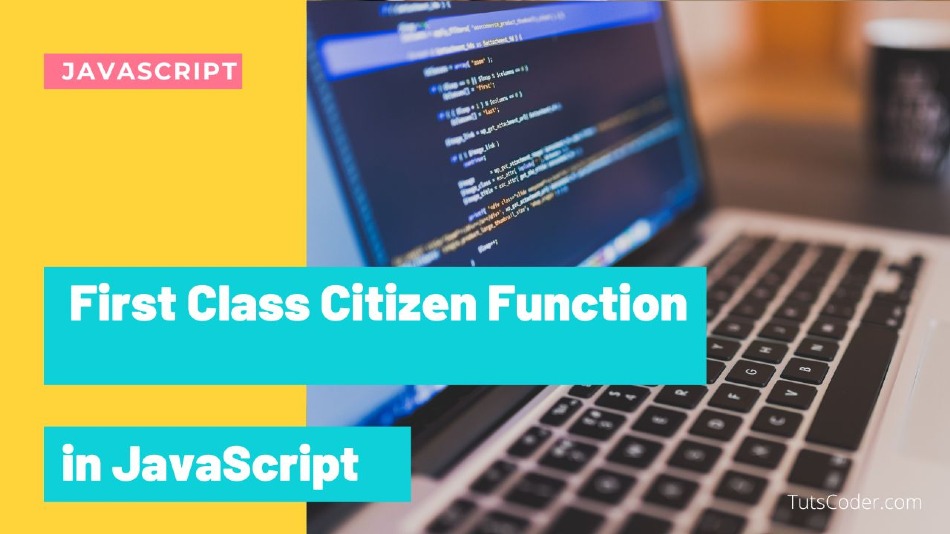
First Class Citizen Function in JavaScript
Functions are treated as first-class citizens in JavaScript. This is because functions are objects in functional programming languages like JavaScript.
What is Functional Programming?
Functional Programming is a form of programming in which you can pass functions as parameters to other functions and also return them as values.
First-Class Functions
The function is first-class citizens of javascript
A programming language is said to have first-class functions when functions in that language are treated like any other variable
In JavaScript, functions are a special type of object. They are Function objects.
Let's see an example,
function greeting() {
console.log('Hello World');
}
// Invoking the function
greeting(); // prints 'Hello World'
We can add properties to functions as we do with objects, as follow
greeting.lang = 'English';
console.log(greeting.lang);
// Output 'English'
Note: Above code is valid, but this is not good practice to assign the object to a function, instead use the separate object.
Everything that can be done with other types like objects, text, or numbers in JavaScript can also be done with functions. They can be assigned to variables, passed around, passed as parameters to other functions (callbacks), etc. For this reason, JavaScript functions are referred to as First-Class Functions.
Assign a function to a variable:
In javascript, we can assign a function to a variable
const thnkyou = function(){
consoloe.log('thank);
}
thnkyou();
Pass a function as an argument:
Since functions are first-class citizens in javascript, we are able to pass them.
const myName = () => {
return "Hello";
};
const greeting = (sayHi, name) => {
console.log(sayHi() + name);
};
greeting(myName, "Jigar");
//OutPut : HelloJigar
Returning functions:
Key concepts of functional programming
function a() {
return function b() {
console.log("This is function B");
};
}
a()();
//Output : This is function B
We can also call this way,
const c = a();
c()
So, that's why we can call first-class citizens of JavaScript.