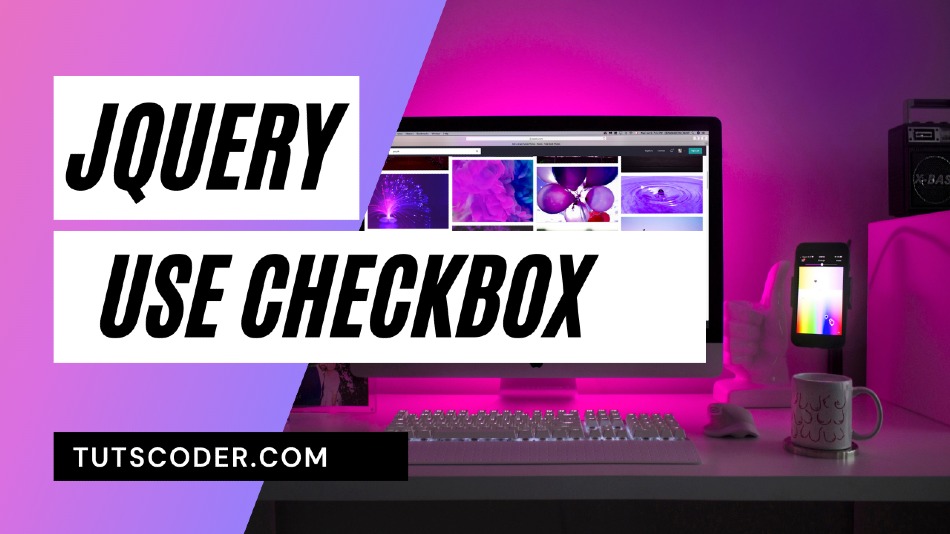
How to check-uncheck all checkbox With jQuery
Howdy Coders,
In this post, we are going to see how to check-uncheck all checkboxes using jQuery.
It becomes useful when you want to allow users to select or deselect all checkboxes at once by clicking one checkbox rather than selecting one by one checkbox Just like Amazon, Flipkart like size uses for its product filtering.
Steps by step Guide to select/Deselect checkbox using jQuery:
If you are new to Jquery or want to learn some basic before starting this tutorial, I must recommend to please checkout learn-javascript-from-scratch and jquery-step-by-step-tutorial-for-beginners
So, here I am going to show how this can be done by using Jquery. It can become handy and I have also used it on many projects and it's working great for me.
So let’s start.
STEP-1:
First of all, you have to add the jquery library to your page:
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script>
</head>
STEP-2:
Create a Blank HTML file and put the following code:
<body>
<div id="container">
<div id="body">
<div class="header" >Check/Uncheck all checkbox with Jquery</div>
<table class="bordered" >
<tr>
<th width="10%"><input type="checkbox" name="chk_all" class="chk_all"></th>
<th >Select All Items</th>
</tr>
<tr>
<td ><input type="checkbox" name="country_id" class="checkboxes" value="6" ></td>
<td > Item-1 </td>
</tr>
<tr>
<td ><input type="checkbox" name="country_id" class="checkboxes" value="7" ></td>
<td > Item-2 </td>
</tr>
<tr>
<td ><input type="checkbox" name="country_id" class="checkboxes" value="8" ></td>
<td > Item-3 </td>
</tr>
<tr>
<td ><input type="checkbox" name="country_id" class="checkboxes" value="9" ></td>
<td > Item-4 </td>
</tr>
<tr>
<td><input type="checkbox" name="country_id" class="checkboxes" value="10" ></td>
<td > Item-5 </td>
</tr>
<tr>
<td ><input type="checkbox" name="country_id" class="checkboxes" value="10" ></td>
<td > Item-6 </td>
</tr>
</table>
</div>
</div>
</body>
STEP-3:
After ending the HTML tag add some Javascript code including with script tag as follow:
<script type="text/javascript">
$(document).ready(function () {
//To Check all checkbox
$(".chk_all").click(function () {
var checkAll = $(".chk_all").prop('checked');
if (checkAll) {
$(".checkboxes").prop("checked", true);
} else {
$(".checkboxes").prop("checked", false);
}
});
// if all checkbox are selected, check the chk_all checkbox
$(".checkboxes").click(function ()
{
if($(".checkboxes").length==$(".subscheked:checked").length)
{
$(".chk_all").attr("checked", "checked");
}
else
{
$(".chk_all").removeAttr("checked");
}
});
});
</script>
Now you are done. If you find any issue checkout the complete source code given below:
Complete source code:
Index.html
<html>
<head>
<script type="text/javascript" src="jquery.js"></script>
<link rel="stylesheet" href="style.css" type="text/css" />
</head>
<body>
<div id="container">
<div id="body">
<div class="header" >Check/Uncheck all checkbox with Jquery</div>
<table class="bordered" >
<tr>
<th width="10%"><input type="checkbox" name="chk_all" class="chk_all"></th>
<th >Select All Items</th>
</tr>
<tr>
<td ><input type="checkbox" name="country_id" class="checkboxes" value="6" ></td>
<td > Item-1 </td>
</tr>
<tr>
<td ><input type="checkbox" name="country_id" class="checkboxes" value="7" ></td>
<td > Item-2 </td>
</tr>
<tr>
<td ><input type="checkbox" name="country_id" class="checkboxes" value="8" ></td>
<td > Item-3 </td>
</tr>
<tr>
<td ><input type="checkbox" name="country_id" class="checkboxes" value="9" ></td>
<td > Item-4 </td>
</tr>
<tr>
<td><input type="checkbox" name="country_id" class="checkboxes" value="10" ></td>
<td > Item-5 </td>
</tr>
<tr>
<td ><input type="checkbox" name="country_id" class="checkboxes" value="10" ></td>
<td > Item-6 </td>
</tr>
</table>
</div>
</div>
</body>
</html>
<script type="text/javascript">
$(document).ready(function () {
//To Check all checkbox
$(".chk_all").click(function () {
var checkAll = $(".chk_all").prop('checked');
if (checkAll) {
$(".checkboxes").prop("checked", true);
} else {
$(".checkboxes").prop("checked", false);
}
});
// if all checkbox are selected, check the chk_all checkbox
$(".checkboxes").click(function ()
{
if($(".checkboxes").length==$(".subscheked:checked").length)
{
$(".chk_all").attr("checked", "checked");
}
else
{
$(".chk_all").removeAttr("checked");
}
});
});
</script>
CSS:
html, body {
margin:0;
padding:0;
height:100%;
}
body {
border: 0;
color: #000;
}
#body{
width: 500px;
margin: 0 auto;
max-width: 100%;
padding:20px 0 70px 0;
height: 100%;
}
#container {
min-height:100%;
position:relative;
}
.header{
font-size: 25px;
text-align: center;
background-color: #d0dafd;
}
table {
*border-collapse: collapse;
border-spacing: 5px;
width: 100%;
}
.bordered {
border: solid darkgoldenrod 3px;
box-shadow: 0 1px 1px darkgoldenrod;
}
.bordered td {
padding: 10px;
border-bottom: 2px solid #d0dafd;
}
.bordered th {
padding: 10px;
border-bottom: 2px solid darkgoldenrod;
background-color: #eee;
border-top: none;
text-align:center;
}
.bordered tbody tr:nth-child(even) {
background: #eeeeee;
border:1px solid #000;
}
.bordered tbody tr:hover td {
background: #d0dafd;
color: #FF0000;
}
tr td {
text-align: center;
}
Conclusion:
Thanks for reading.
Do let me know If you face any difficulties please feel free to comment below we love to help you. if you have any feedback suggestions then please inform us by commenting.
Don’t forget to share this tutorial with your friends on Facebook and Twitter.