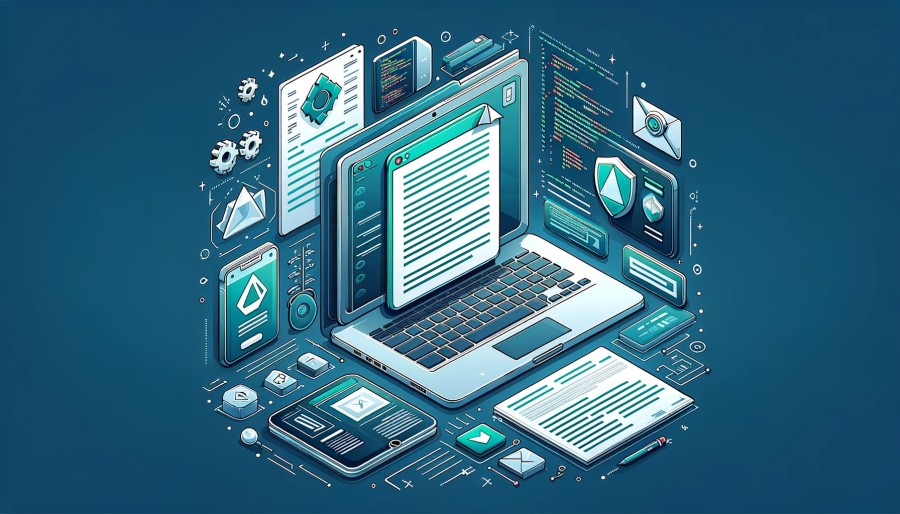
How to Generate PDF in Angular App: A Step-by-Step Guide
PDF generation is a powerful feature for Angular applications, allowing users to download receipts, reports, or even certificates directly from your app. In this guide, we’ll learn how to generate high-quality PDFs in an Angular app using the popular html2pdf.js library.
This step-by-step tutorial will walk you through setting up, generating, and customizing PDFs. We'll also use real-life scenarios like creating invoices to make the process relatable and practical. Let’s dive in! 🎉
Table of Contents
- Why Use PDF Generation in Angular Apps?
- What is html2pdf.js?
- Setting Up the Angular Project
- Step-by-Step PDF Generation
- 1. Design Your HTML Template
- 2. Add CSS for a Professional Look
- 3. Add PDF Generation Logic
- 4. Add a Button to Trigger the Download
- Real-World Use Case Example
- Why Choose html2pdf.js for Angular PDF Generation?
- Final Thoughts
Why Use PDF Generation in Angular Apps?
Adding PDF generation to your app can enhance its functionality significantly:
- 📄 Invoices: Allow customers to download their invoices directly from the app.
- 📊 Reports: Export analytical reports for your users.
- 🎓 Certificates: Let learners download their course completion certificates.
- 🛍️ Receipts: Provide downloadable receipts for online orders.
For example, imagine you’re building an e-commerce app. With PDF export, users can easily download receipts or share order details with others!
What is html2pdf.js?
html2pdf.js is a popular JavaScript library that combines html2canvas and jspdf to capture web content as an image and convert it into a PDF. It’s simple to use and highly customizable, making it a great choice for Angular developers.
Key Features:
✅ Converts HTML content into PDFs.
✅ Supports CSS styles for polished designs.
✅ Offers built-in download functionality.
Setting Up the Angular Project
Let’s start by setting up an Angular app. If you already have one, you can skip this step.
ng new angular-pdf-app
cd angular-pdf-app
npm install html2pdf.js
Step-by-Step PDF Generation
1. Design Your HTML Template
Create a component with an HTML template for your content. Here’s an example for generating an invoice:
Invoice 🧾
Invoice #: {{ invoice.id }}
Date: {{ invoice.date }}
Customer Name: {{ invoice.customerName }}
Item
Quantity
Price
{{ item.name }}
{{ item.quantity }}
{{ item.price }}
Total: {{ invoice.total }}
This template dynamically updates with Angular bindings, making it ideal for real-time data like invoices or reports.
2. Add CSS for a Professional Look
Style your content to make the PDF look polished. For example:
.invoice {
padding: 20px;
border: 1px solid #ddd;
width: 800px;
margin: 0 auto;
font-family: Arial, sans-serif;
}
table {
width: 100%;
border-collapse: collapse;
margin-top: 20px;
}
th, td {
border: 1px solid #ddd;
padding: 10px;
text-align: left;
}
h1, h3 {
text-align: center;
}
3. Add PDF Generation Logic
In your component, use the html2pdf.js library to generate and download the PDF.
import { Component, ElementRef, ViewChild } from '@angular/core';
import * as html2pdf from 'html2pdf.js';
@Component({
selector: 'app-invoice',
templateUrl: './invoice.component.html',
styleUrls: ['./invoice.component.css']
})
export class InvoiceComponent {
@ViewChild('invoiceContent') invoiceContent!: ElementRef;
invoice = {
id: '12345',
date: new Date().toLocaleDateString(),
customerName: 'John Doe',
items: [
{ name: 'Product A', quantity: 2, price: '$20' },
{ name: 'Product B', quantity: 1, price: '$50' },
],
total: '$90'
};
generatePDF(): void {
const content = this.invoiceContent.nativeElement;
const options = {
margin: 1,
filename: `invoice_${this.invoice.id}.pdf`,
image: { type: 'jpeg', quality: 0.98 },
html2canvas: { scale: 2 },
jsPDF: { unit: 'in', format: 'letter', orientation: 'portrait' }
};
html2pdf().set(options).from(content).save();
}
}
This method:
- Captures the HTML content.
- Converts it into a PDF.
- Automatically downloads it with the specified filename.
4. Add a Button to Trigger the Download
Add a button in your template to call the generatePDF
method:
When clicked, the invoice will be converted into a downloadable PDF! 🎉
Real-World Use Case Example
Imagine you're building a course management app where students can download their certificates upon completion. By replacing the invoice template with a certificate design, you can easily generate customized PDFs for each student.
Why Choose html2pdf.js for Angular PDF Generation?
html2pdf.js is lightweight, flexible, and easy to implement. Its combination of html2canvas and jspdf ensures high-quality PDFs with minimal effort.
Final Thoughts
Generating PDFs in an Angular app is no longer a daunting task! With html2pdf.js, you can provide users with dynamic, professional-quality documents for a variety of use cases. Whether you’re building an invoice generator, an analytics exporter, or a certificate generator, this library has you covered.
Want to learn more about enhancing your Angular app? Check out authoritative resources like Angular's official site. Let us know how you’re using PDF generation in your project!
💡 Pro Tip: Share this guide with your fellow Angular developers to help them level up their apps! 🎯