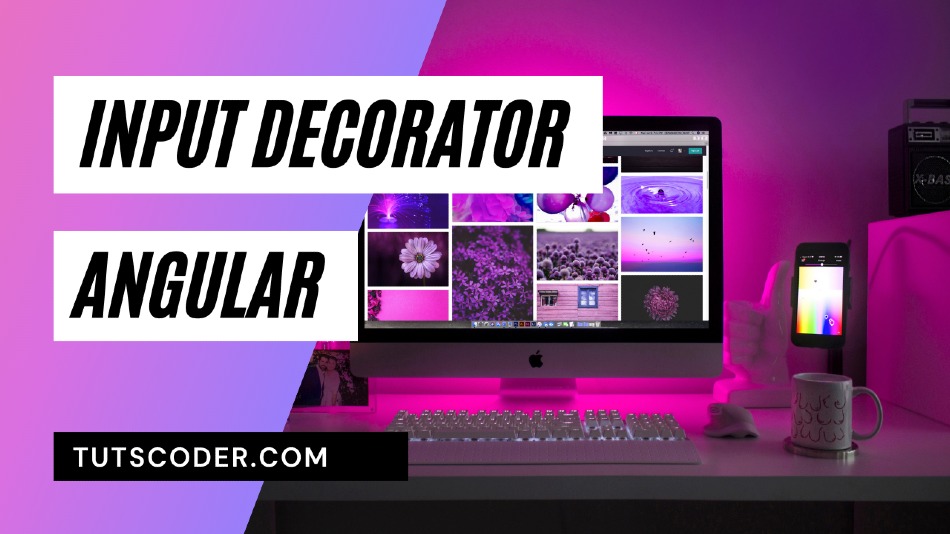
How to use @input Decorator in Angular 14+
In this tutorial, we will learn how to use Input decorator in angular and using this we can pass data from parent component to child component.
So let’s get started…
What is an Input decorator?
Input decorator is used to creating custom properties, It makes available properties for other components.
Also read, How to Use @Output Decorator in Angular
Passing Data from Parent to Child Component in Angular using @input decorator Step-by-Step :
Step 1: Create New Project
Create Blank Project in angular using below command, Skip this step if you have already:
ng new angularDemo
Step 2: Add Components
Now add two new components with name parent and child component, using below command
ng new g c parent
ng new g c child
Step 3: Add parent Component
To render our parent component HTML we need to include it to app.component.html file as HTML tag as shown below:
<div style="text-align:center">
<h1>
Welcome to {{ name }}!
</h1>
<hr>
<app-parent></app-parent>
</div>
Step 4: HTML for Parent and Child Component
Now suppose we have a scenario where we have to change the price from parent component and it should be effect on child component, so let’s do HTML for this as below:
HTML for parent component:
<h1>
Parent component
</h1>
<input type="text"/>
<app-child></app-child>
HTML for child component:
<h1>
Child component
</h1>
<h4>
Current Price : {{newPrice}}
</h4>
In Parent HTML code we have added <app-child> tag to use it as child component.
Step 5: Pass data using Input decorator
Now, to make able child component to receive value from parent component we need to use Input decorator as follows in child.component.ts, see below code:
import { Component, OnInit, Input } from '@angular/core';
@Component({
selector: 'app-child',
templateUrl: './child.component.html',
styleUrls: ['./child.component.css']
})
export class ChildComponent implements OnInit {
constructor() { }
@Input() public newPrice: string = '500';
ngOnInit() {
}
}
Step 6: Reference to the child component value
Next, we need to make reference to the child component value, so update HTML as below in parent.component.ts
as below:
<input type="text" #price (keyup)="onchange(price.value)"/>
<app-child [newPrice]="currentPrice"></app-child>
Now our Application is ready, run application using ng serve command then go to a browser it will look like this:
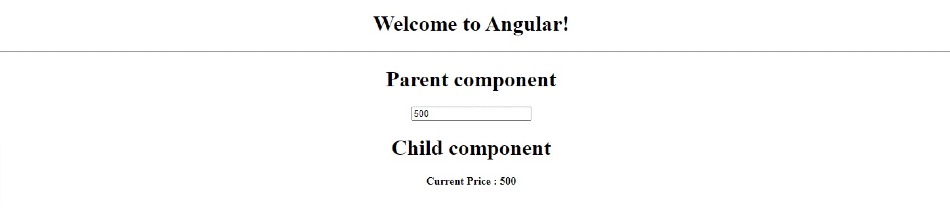
Now you can see when you update any value from parent component input it will also change on child component.
So, using Input decorator we can pass data from the parent component to the child component.