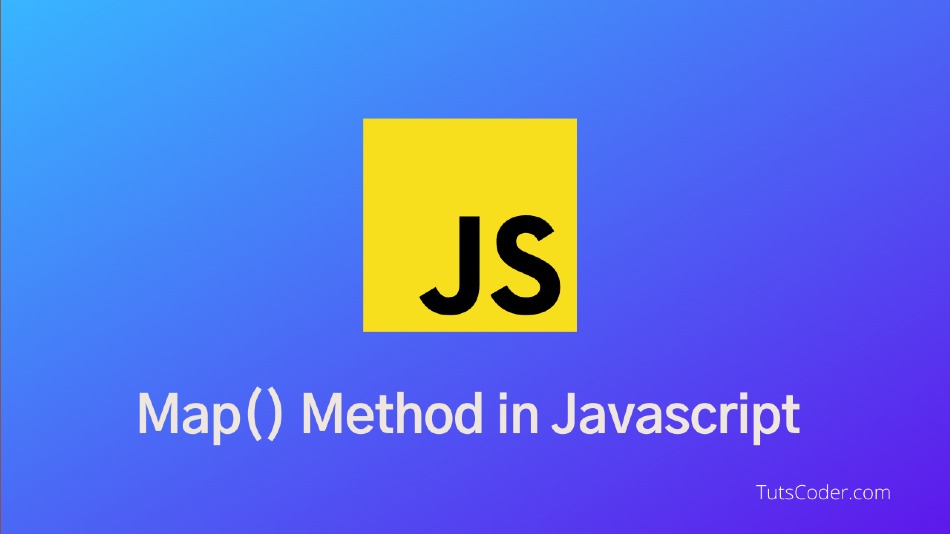
Introduction to map() Method in JavaScript
In this tutorial, we will learn about how to use and when to use the Array Map method in JavaScript.
In Javascript, there are three big and important array methods that we use all the time to perform data transformation.
The Array.map() method allows you to iterate over an array and modify its elements using a callback function.
We can use Map method to loop over array Similar to forEach method
Main diffrence between Map and foreach is map map creates new array based on the original array
map returns a new array containing the resultsof appliying an operation on all original array elements
For Example:
suppose you have the following array element:
let arr = [2, 1, 7, 3];
Now you wan't to multiply each of the array's elements by 5.
We can do this by using map method as below:
let arr = [2, 1, 7, 3];
let modifiedArr = arr.map(function(element){
return element *5;
});
console.log(modifiedArr); // [10, 5, 35, 15]
We can Also simplifies this using arrow function as follows:
//Using arrow function
let modifiedArr = arr.map(ele=> ele* 5);
console.log(modifiedArr); // [10, 5, 35, 15]
So, using the Array.map() method we can apply some changes to the elements, whether multiplying by a specific number as shown in the code above or doing any other operations that you might require for your application
How to use map() over an array of objects
For example, supposr you have an array of objects that have firstName and lastName values of users as follows
let users = [
{firstName : "Ali", lastName: "Mehta"},
{firstName : "Joe", lastName: "Doe"},
{firstName : "Steve", lastName: "Smith"}
];
Now you wants the array with join of firstName and lastName, then you can use map maethod as below:
let userFullnames = users.map(function(element){
return `${element.firstName} ${element.lastName}`;
})
console.log(userFullnames);
// ["Ali Mehta", "Joe Doe", "Steve Smith"]
How to get index of element using map()
We can get index of element using map() as follows:
//Use index with map
let users = [
{firstName : "Ali", lastName: "Mehta"},
{firstName : "Joe", lastName: "Doe"},
{firstName : "Steve", lastName: "Smith"}
];
let userData = users.map((element,index)=>
`${index + 1} : ${element.firstName} ${element.lastName}`);
console.log(userData );
// ["1 : Ali Mehta", "2 : Joe Doe", "3 : Steve Smith"]
Conclusion:
Do let me know If you face any difficulties please feel free to comment below we love to help you.
If you have any feedback suggestion then please inform us by commenting.
Don’t forget to share this tutorial with your friends on Facebook and Twitter