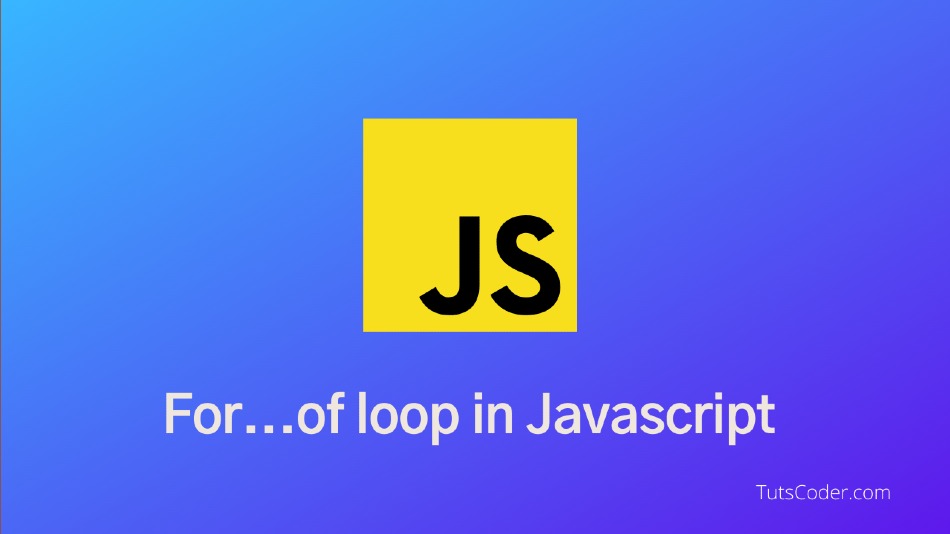
Introduction to JavaScript for…of Loop in ES6
In this tutorial, we will learn about for of loop in javascript.
So let's get started...
What is the For...of Loop?
For...of loop was introduced in ES6 which iterates over iterable such as Arrays, strings, Maps, Sets, DOM collections,and more.
Looping through Array:
const colors= ['red', 'green'];
for (const color of colors) {
console.log(color);
}
// 'red'
// 'green'
The array method entries() can be used to access the index of the iterated item.
//Getting Index with array element
const colors= ['red', 'green'];
for (const [index, color] of colors.entries()) {
console.log(index, color);
}
// 0, 'red'
// 1, 'green'
Iterate plain JavaScript objects:
const user= {
name: 'Ankit',
job: 'developer'
};
for (const [property, value] of Object.entries(user)) {
console.log(property, value);
}
// 'name', 'Ankit'
// 'job', 'developer'
Iterate DOM collections
HTMLCollection is an array-like object instead of a regular array, so you don’t have access to regular array methods.
In JavaScript, the children property of every DOM element is an HTMLCollection. So, using for...of
we can also iterate over array-like objects,as below:
const children = document.body.children;
for (const child of children) {
console.log(child); // will logs each child of <body>
}
In addition, for...of can also iterate over NodeList collections which are iterables.
For example, the function document.querySelectorAll(query) returns a NodeList:
const images= document.querySelectorAll('img');
for (const image of images) {
console.log(image); // log each image in the document
}
Conclusion:
Do let me know If you face any difficulties please feel free to comment below we love to help you.
If you have any feedback suggestion then please inform us by commenting. Don’t forget to share this tutorial with your friends on Facebook and Twitter