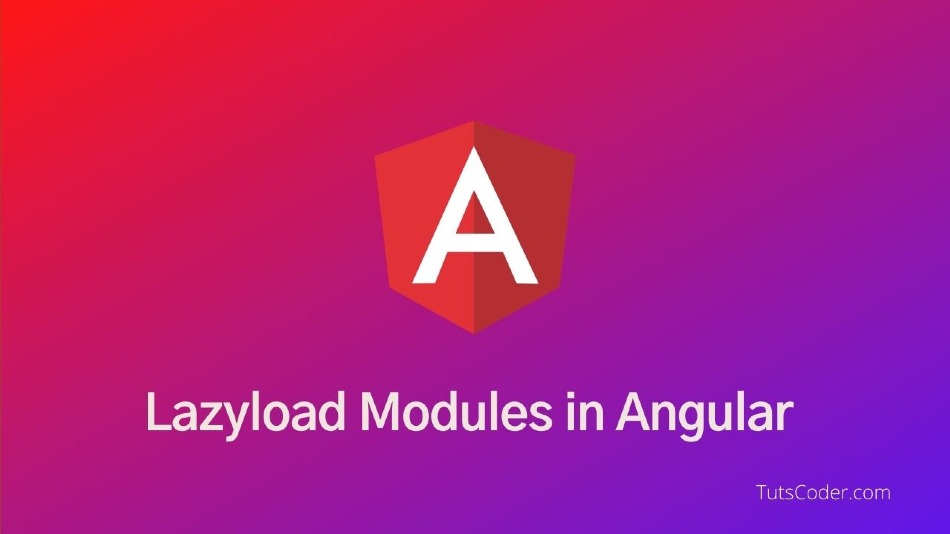
How to Lazy Load Modules in Angular 14 with Dynamic Imports
In this tutorial, we will learn how to lazy load modules in Angular 14 using dynamic imports.
What is Lazy Loading?
Lazy loading is the way to download only when it requires or in chunks instead of bigger pieces.
In this tutorial, we will use dynamic imports and loadChildren properties to achieve lazy loading in Angular.
Also read, 5 Reasons Angular Is The Next Big Thing In Web Development
Create Angular Application
Run the following command to install the latest version of Angular CLI:
npm install -g @angular/cli
Install a new angular application:
ng new angular-lazy-load-sample
Navigate to the project root:
cd angular-lazy-load-sample
Generate Angular Module
Lazy loading is entirely dependent on modules, so execute the below command from the command prompt to generate a module:
ng generate module user
Add two-component inside user module using below command:
ng generate component user/list
ng generate component user/user-details
Lazy Load with LoadChildren
By using loadChildren property, we can lazy load modules as follows:
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
const routes: Routes = [
{
path: 'user',
loadChildren: () => import(`./user/user.module`).then(
module => module.UserModule
)
},
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
Setting Up Routes
Here, we need to create a separate user routing, which will handle the lazy loading for the components associated with it.
ng g m user/user --routing
Now, import the components to blog module and pass them in routes array.
Then go to the user/user-routing.module.ts file and add the following code:
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { ListComponent } from '../list/list.component';
import { UserDetailsComponent } from '../user-details/user-details.component';
const routes: Routes = [
{ path: '', component: ListComponent },
{ path: 'user-details', component: UserDetailsComponent }
];
@NgModule({
imports: [RouterModule.forChild(routes)],
exports: [RouterModule]
})
export class UserRoutingModule { }
Above we have defined the routes for list and User Details components within the UserRoutingModule.
Next, go to user.module.ts and place the following code:
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { UserRoutingModule } from './user/user-routing.module';
import { ListComponent } from './list/list.component';
import { UserDetailsComponent } from './user-details/user-details.component';
@NgModule({
imports: [
CommonModule,
UserRoutingModule
],
declarations: [ListComponent, UserDetailsComponent]
})
export class UserModule { }
Now its done, our application lazy-loaded successfully, you can check it by navigating to network tab in developer tools.
Conclusion:
Do let me know If you face any difficulties please feel free to comment below we love to help you.
If you have any feedback suggestion then please inform us by commenting.
Don’t forget to share this tutorial with your friends on Facebook and Twitter