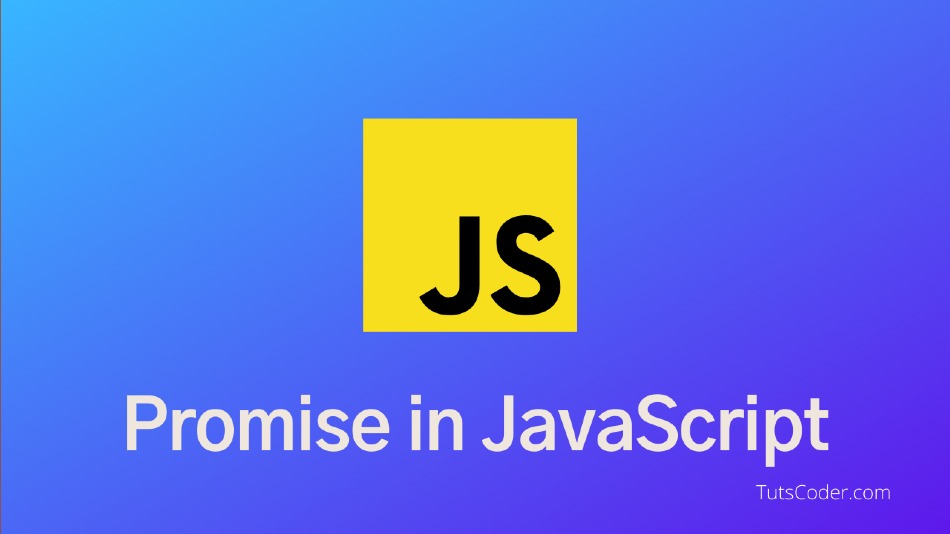
JavaScript Promises Demystified: A Step-by-Step Tutorial for Beginners
Asynchronous programming is an essential aspect of modern web development, and JavaScript Promises offer an elegant solution for managing asynchronous operations. If you're new to Promises and find them confusing, worry no more! In this tutorial, we'll demystify JavaScript Promises, providing a beginner-friendly guide accompanied by practical examples.
What is a Promise?
Promises are an ES6 feature and they became available in JavaScript in 2015.
Promise is an object that is used basically as a placeholder for the future result of an asynchronous operation.
we can also say that a promise is like a container for a future value.
Using promises we can escape callback hell.
Promises in JavaScript
Promises are essentially just a special kind of object in JavaScript.
we usually only built promises to basically wrap old callback-based functions into promises and this is a process that we call promisifying.
So basically promisifying means to convert callback-based asynchronous behavior to promise-based.
Now, since promises work with asynchronous operations, they are time sensitive,so they change over time.
How Promise works?
Promises can be in different states and this is what they call the cycle of a promise.
So in the very beginning, we say that a promise is pending and so this is before any value resulting from the asynchronous task is available.
Now, during this time, the asynchronous task is still doing its work in the background.
Then when the task finally finishes, we say that the promise is settled and there are two different types of settled promises and that's fulfilled promises and rejected promises.
So a fulfilled promise is a promise that has successfully resulted in a value just as we expect it.
For example, when we use the promise to fetch data from an API, a fulfilled promise successfully gets that data, and it's now available to being used.
On the other hand, a rejected promise means that there has been an error during the asynchronous task.
And the example of fetching data from an API,an error would be for example, when the user is offline and can't connect to the API server.
There are 3 states of the Promise object:
- Pending: Initial State, before the Promise succeeds or fails
- Resolved: Completed Promise
- Rejected: Failed Promise
Also read, Promises helper functions
Building a Simple Promise
we can create a new promise using the promise constructor, as shown below.
const myPromise = new Promise();
It takes two parameters, one for success (resolve) and one for fail (reject):
const myPromise = new Promise((resolve, reject) => {
// condition
});
Finally, there will be a condition. If the condition is met, the Promise will be resolved, otherwise, it will be rejected:
const myPromise = new Promise((resolve, reject) => {
let condition;
if(condition is met) {
resolve('Promise is resolved successfully.');
} else {
reject('Promise is rejected');
}
});
So we have created our first Promise. Now let's use it.
then( ):
The then( ) method is called after the Promise is resolved.
For example, let’s log the message to the console that we got from the Promise:
myPromise.then((message) => {
console.log(message);
});
catch( ):
We can use the catch() method to handle failed or rejected promises, see below example:
myPromise.then((message) => {
console.log(message);
}).catch((message) => {
console.log(message);
});
So if the promise gets rejected, it will jump to the catch( ) method
finally( ):
Now the finally method is not always useful, but sometimes it actually is.
So we use this method for something that always needs to happen
no matter the result of the promise, And one good example of that is to hide a loading spinner
myPromise.then((message) => {
if (message!== ‘hello’){
throw new Error(“Oops, you didn’t type hello”)
}
}).catch((message) => {
console.log(message);
},finally {
alert(‘thanks for visiting!’)
});
whenever we want to create some error that we want to handle down here,
in the catch handler, all we need to do is to throw, because throwing an error inside of this callback function of this then method will immediately reject this promise.
Conclusion:
Congratulations! You've successfully demystified JavaScript Promises through our step-by-step tutorial for beginners. By understanding Promises and their practical usage, you're equipped to write efficient and reliable asynchronous code. Embrace Promises in your JavaScript projects and unlock a world of improved performance and maintainability.
Do let me know If you face any difficulties please feel free to comment below we love to help you.
If you have any feedback suggestion then please inform us by commenting.
Don’t forget to share this tutorial with your friends on Facebook and Twitter