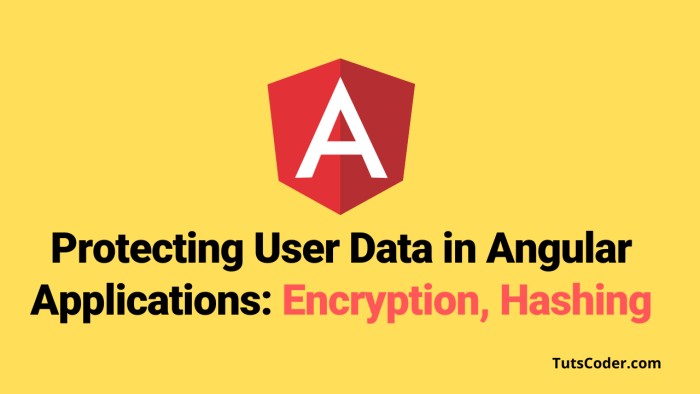
Protecting User Data in Angular Applications: Encryption, Hashing, and More
As an Angular developer, one of your top priorities should be to protect user data. With the increasing number of cyber-attacks and data breaches, it's essential to implement robust security measures to safeguard your users' information. In this blog post, we'll explore some of the best practices for protecting user data in Angular applications, including encryption, hashing, and other techniques.
What is Encryption?
Encryption is the process of converting plaintext (i.e., readable data) into an unreadable form known as ciphertext. Encryption helps to protect sensitive data by making it difficult for unauthorized parties to access or interpret the information. In Angular applications, you can use various encryption algorithms, such as AES, RSA, and Blowfish.
For example, let's say you have a user's credit card information stored in your Angular application. Instead of storing the credit card number in plain text, you can encrypt it using AES encryption. That way, even if an attacker gains access to the database, they won't be able to read the credit card information without the encryption key.
What is Hashing?
Hashing is the process of transforming data into a fixed-length string of characters known as a hash. Hashing is often used to store passwords securely. When a user creates an account and sets a password, the password is hashed and stored in the database. When the user logs in, the entered password is hashed and compared to the stored hash. If the hashes match, the user is granted access.
Angular provides a built-in crypto module that you can use for hashing data. For example, here's how you can hash a password in Angular:
import { createHash } from 'crypto';
const hash = createHash('sha256');
hash.update('password123');
const hashedPassword = hash.digest('hex');
Other Techniques for Protecting User Data
In addition to encryption and hashing, there are other techniques you can use to protect user data in Angular applications. Some of these techniques include:
- Two-factor authentication: This involves requiring users to provide two forms of identification (e.g., a password and a code sent to their phone) before granting access to their account.
- Input validation: This involves ensuring that user input is in the expected format and is within acceptable ranges. Input validation can help prevent attacks such as SQL injection and cross-site scripting (XSS).
- Regular security audits: Regular security audits can help identify vulnerabilities and weaknesses in your application's security measures. You can use tools such as OWASP ZAP to perform automated security scans.
Conclusion
Protecting user data in Angular applications is crucial for maintaining user trust and complying with data protection regulations. In this blog post, we've covered some of the best practices for protecting user data, including encryption, hashing, and other techniques. By implementing these techniques and staying up-to-date with the latest security trends, you can help ensure that your Angular application is secure and reliable.