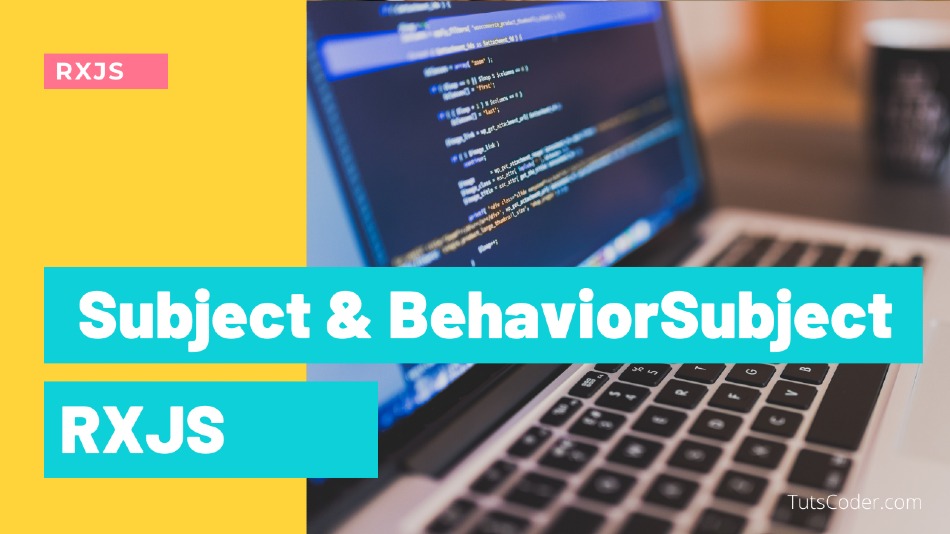
What is Subject and BehaviorSubject - RXJS
In this tutorial, we will learn about the subject and BehaviorSubject of RXJS.
What is a Subject?
A Subject is a particular type of Observable from the RxJS library.
The subject is mostly used for cross-component data share/Communication.
The subject is a special type of observable because subject works as observable and observer also.
We can do gloabaly data communication using subject
The subject is Multicasting observable means we will get data from when we subscribed not previous data.
Types of Subjects
- Subject
- ReplaySubject
- BehaviorSubject
- AsyncSubject
A subject has three methods which you can use:
- subscribe() : with this method, you can activate the subscription of a new subscriber.
- next(): with this method, you can pass new values. All the current subscribers will receive this.
- complete(): with this method, you close all the subscriptions to the Subject
Also note that a Subject doesn't have an initial value. Every value passed with the next method will send the values to all the subscribers.
Example:
import { Injectable } from '@angular/core';
import { Subject } from 'rxjs';
@Injectable()
export class DataService {
username = new Subject <string>("Jigar");
constructor() { }
updatedDataSelection(data){
this.username.next(data);
}
}
We can call next and pass in a new value to the Subject.
import { Component, OnInit } from "@angular/core";
import { DataService } from "./data.service";
@Component({
selector: "app-header",
templateUrl: "./header.component.html",
})
export class HeaderComponent implements OnInit {
constructor(private dataService : DataService ) {}
exclusive = false;
ngOnInit() {
this.dataService.username.next('rahul');
}
}
For getting the updated value we can subscriber observable as below:
import { Component, OnInit } from "@angular/core";
import { DataService } from "./data.service";
@Component({
selector: "app-header",
templateUrl: "./header.component.html",
})
export class HeaderComponent implements OnInit {
constructor(private dataService : DataService ) {}
exclusive = false;
ngOnInit() {
this.dataService.exclusive.subscribe((res) => {
console.log(res):
});
}
}
When to use:
When you need multiple subscribers and care that all the subscribers are getting their new values simultaneously, use Subject.
BehaviorSubject
A BehaviorSubject a Subject that can emit the current value while Subjects have no concept of current value.
Example:
import { Injectable } from '@angular/core';
import { BehaviorSubject } from 'rxjs/BehaviorSubject';
@Injectable()
export class DataService {
username = new BehaviorSubject<string>("Jigar");
constructor() { }
updatedDataSelection(data){
this.username.next(data);
}
}