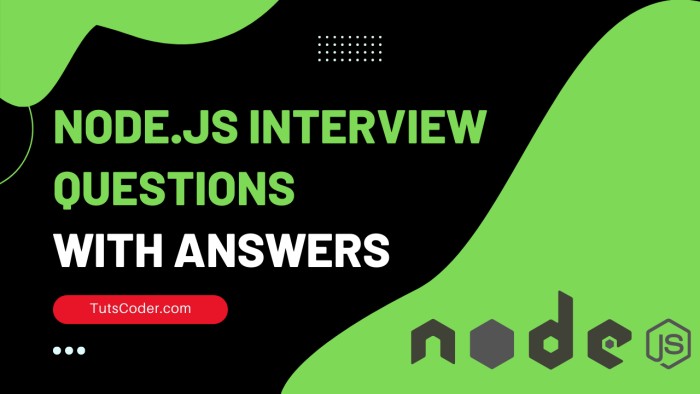
Top 29 Node.js Interview Questions with Answers and Examples
Are you preparing for a Node.js interview? Whether you're a beginner or an experienced developer, it's always helpful to have a collection of common interview questions and answers to review. In this blog post, we'll cover 29 frequently asked Node.js interview questions and provide detailed answers and examples to help you ace your interview.
Q: Why would you choose to use Node.js for developing an application?
A: There are several reasons to choose Node.js for application development:
- Asynchronous and Non-blocking: Node.js uses an event-driven, non-blocking I/O model, which allows it to handle concurrent requests efficiently.
- Scalability: Node.js is designed to handle a large number of concurrent connections with minimal resources, making it suitable for building highly scalable applications.
- JavaScript Everywhere: Node.js enables developers to use JavaScript on both the client and server, allowing for code reuse and a unified development experience.
- Rich Ecosystem: Node.js has a vast ecosystem of open-source libraries and modules available through npm, making it easy to find and integrate third-party functionality into your application.
- Real-time Applications: Node.js is well-suited for building real-time applications, such as chat, gaming, collaboration tools, and streaming platforms, thanks to its event-driven architecture and WebSocket support.
Q: What are the advantages of using Node.js?
A: Node.js offers several advantages, making it a popular choice for server-side development. Some key advantages of using Node.js include:
- Asynchronous and Non-Blocking: Node.js uses an event-driven, non-blocking I/O model, allowing it to handle high concurrency with excellent performance and scalability.
- JavaScript Everywhere: Node.js uses JavaScript, making it possible to use the same language both on the front-end and back-end, simplifying development and code reuse.
- Vast Ecosystem: Node.js has a rich ecosystem with a wide range of libraries, frameworks (like Express), and tools that streamline development and provide ready-to-use solutions for common tasks.
- Fast Execution: The V8 JavaScript engine used by Node.js provides fast execution and performance optimizations, resulting in efficient code execution.
- Community and Support: Node.js has a vibrant and active community, providing extensive support, documentation, and a vast collection of open-source modules.
- Microservices and Scalability: Node.js is well-suited for building microservices architectures and scalable applications, allowing independent development and deployment of small, focused services.
- Real-Time Applications: Node.js excels in building real-time applications like chat apps, collaborative tools, or live streaming platforms, thanks to its event-driven nature and WebSocket support.
Q: Can you explain the architecture of Node.js?
A: Node.js follows a modular and event-driven architecture. Here's a high-level overview of the Node.js architecture:
- V8 JavaScript Engine: Node.js is built on the V8 JavaScript engine, which provides the runtime environment for executing JavaScript code.
- Event Loop: Node.js utilizes an event loop to handle concurrent requests and I/O operations asynchronously. The event loop continuously checks for pending events and executes their associated callback functions.
- Libuv: Node.js utilizes the Libuv library, which provides an event-driven, cross-platform I/O framework that abstracts the underlying operating system's I/O capabilities.
- Core Modules: Node.js includes a set of core modules (e.g., http, fs, path) that provide essential functionalities for building web servers, working with file systems, handling network communication, etc.
- NPM: Node Package Manager (NPM) is the default package manager for Node.js, allowing developers to easily install, manage, and share reusable code modules (packages) from the NPM registry.
- Module System: Node.js uses a CommonJS-based module system, allowing developers to organize their code into reusable modules and manage dependencies between modules using require and module.exports or exports.
- Third-Party Modules: Node.js has a vast ecosystem of third-party modules available through NPM, providing additional functionalities and integrations with various libraries, frameworks, and services.
- Web Frameworks: Node.js web frameworks like Express, Koa, or Hapi provide higher-level abstractions and features to streamline web application development.
- Data Access: Node.js can interact with databases using database-specific drivers (e.g., mysql2, mongodb) or ORM libraries (e.g., Sequelize, Mongoose) for data modeling and abstraction.
- Deployment Options: Node.js applications can be deployed on various platforms, including traditional servers, cloud-based platforms (e.g., AWS, Google Cloud), and serverless architectures (e.g., AWS Lambda).
This is a simplified overview of the Node.js architecture, and the actual implementation details can vary depending on the specific use case and application design.
Q: What is an Error First callback in Node.js?
A: Error First callback is a convention followed in Node.js where the first parameter of a callback function is reserved for an error object. If an error occurs during the asynchronous operation, it is passed as the first argument to the callback. If no error occurs, the first argument is set to null
or undefined
, and the subsequent arguments contain the result of the operation.
Example:
function readFile(callback) {
fs.readFile('file.txt', 'utf8', function(error, data) {
if (error) {
callback(error);
} else {
callback(null, data);
}
});
}
Q: How would you implement a real-time application, like a chat, using Node.js?
A: To implement real-time applications in Node.js, you can utilize the power of WebSockets. WebSockets provide a bidirectional communication channel between the client and server, enabling real-time updates without the need for continuous HTTP polling.
Example: You can use the socket.io
library in Node.js to handle WebSocket communication.
Q: Is there a limit to the number of WebSockets that can be handled by a Node.js server?
A: The number of WebSockets that can be handled by a Node.js server depends on various factors, such as available server resources (CPU, memory, network), the implementation of the WebSocket server, and the configuration of the underlying infrastructure. Node.js is known for its ability to handle a large number of concurrent connections, but it's essential to optimize your code and infrastructure to handle the expected load.
Q: What are microservices and microfrontends, and how are they related to Node.js?
A: Microservices and microfrontends are architectural patterns where an application is broken down into smaller, loosely coupled services or front-end components. Each microservice or microfrontend operates independently and communicates with others through well-defined APIs.
Node.js is often used in microservices and microfrontend architectures due to its lightweight nature, asynchronous capabilities, and ease of building RESTful APIs or GraphQL endpoints. It allows developers to create independent services that can be developed, deployed, and scaled independently, promoting modular and scalable application development.
Q: In which scenarios would you choose to use microfrontends?
A: Microfrontends are a suitable choice when you have a complex front-end application with multiple teams working on different parts of the user interface. It enables independent development and deployment of individual front-end components. Some common scenarios where microfrontends can be beneficial include:
- Large-scale applications: When you have a large application with multiple teams, microfrontends allow independent development and deployment of each section.
- Autonomous teams: If you have teams with specialized expertise (e.g., different UI frameworks, technologies), microfrontends enable them to work independently.
- Continuous delivery: Microfrontends make it easier to achieve continuous delivery by allowing updates to specific sections of the application without affecting the entire system.
However, it's essential to carefully consider the complexity and overhead of managing microfrontends, as it introduces additional operational and coordination challenges.
Q: Why is debugging important in Node.js development?
A: Debugging is a critical part of the development process. It helps identify and fix issues in the code, ensuring the application works as intended. Some reasons why debugging is important in Node.js development include:
- Bugs and Errors: Debugging helps identify and resolve bugs, errors, and unexpected behavior in the code.
- Performance Optimization: Debugging allows developers to analyze performance bottlenecks and optimize code for better performance.
- Understanding Execution Flow: Debugging provides insights into the execution flow of the application, helping developers understand how the code behaves.
- Testing and Validation: Debugging assists in validating the correctness of the code during testing and quality assurance processes.
- Enhancing Reliability: By identifying and fixing issues, debugging enhances the reliability and stability of the application.
Q: Is Node.js single-threaded or does it support multiple threads?
A: Node.js is single-threaded by default. However, it employs an event-driven, non-blocking I/O model, allowing it to handle concurrent requests efficiently. Although Node.js runs on a single thread, it utilizes an event loop and asynchronous operations to maximize CPU utilization and responsiveness.
It's important to note that while the JavaScript code runs on a single thread, Node.js employs a multi-threaded approach for handling I/O operations. I/O operations, such as file system operations and network requests, are delegated to worker threads or handled asynchronously using non-blocking APIs.
Q: How does Node.js handle concurrency if it's single-threaded?
A: Node.js uses an event-driven, non-blocking I/O model to handle concurrency efficiently. Here's a simplified overview of how Node.js handles concurrency:
- Event Loop: Node.js has an event loop that continuously checks for pending I/O operations or events.
- Non-Blocking I/O: When a non-blocking I/O operation (e.g., file read, network request) is initiated, Node.js registers a callback function and continues executing the next operations without waiting for the result.
- Asynchronous Execution: Once the I/O operation is completed, the event loop notifies the associated callback, and it gets executed asynchronously.
- Concurrency with Event Loop: While waiting for I/O operations, Node.js can handle other requests or events, maximizing CPU utilization and concurrency.
By utilizing non-blocking I/O and asynchronous execution, Node.js can handle multiple concurrent operations efficiently on a single thread.
Q: What is the event loop in Node.js, and what are the phases in the event loop?
A: The event loop is a core component of Node.js responsible for handling and dispatching events in an asynchronous, non-blocking manner. It allows Node.js to efficiently handle concurrent requests. The event loop operates in several phases, including:
- Timers: The timers phase handles the callbacks scheduled by setTimeout() and setInterval() functions. It checks if any timers have expired and executes their associated callbacks.
- I/O Callbacks: In this phase, I/O-related callbacks (e.g., network operations, file system access) that were deferred during the previous event loop iteration are executed.
- Idle, Prepare: These are internal phases where Node.js prepares for the next iteration of the event loop.
- Poll: The poll phase retrieves new I/O events from the operating system. If there are no pending I/O events, it will wait for events to occur. If there are callbacks waiting in the poll queue, they are executed immediately.
- Check: The check phase executes callbacks registered with setImmediate().
- Close Callbacks: The close callbacks phase executes callbacks associated with closed resources, such as sockets or file handles.
The event loop continuously iterates through these phases, handling events and executing associated callbacks until there are no more events or callbacks remaining.
It's important to note that the precise behavior of the event loop can be influenced by factors such as the operating system, the presence of worker threads, or the usage of specific APIs or libraries within the application.
Q: How do you manage processes in Node.js?
A: Node.js provides the child_process
module, which allows you to create and manage child processes from your Node.js application. You can use this module to spawn new processes, communicate with them, and handle their execution.
Q: How do you make a database connection in Node.js?
A: To establish a database connection in Node.js, you typically use a database-specific driver or an ORM (Object-Relational Mapping) library. Here's an example using the popular mysql2
library to connect to a MySQL database:
Q: Are you familiar with MySQL?
A: Yes, I'm familiar with MySQL. It is one of the most popular open-source relational databases widely used in web development. MySQL provides a robust, scalable, and high-performance database solution for various applications. It supports SQL (Structured Query Language) for managing and querying data.
MySQL is known for its reliability, ACID compliance, and extensive community support. It offers various features such as replication, clustering, transaction support, and advanced security mechanisms. MySQL can be easily integrated with Node.js using libraries like mysql
, mysql2
, or ORM frameworks like Sequelize or TypeORM.
Q: What is middleware in the context of Node.js?
A: Middleware refers to functions or code that sit between the server and the application's routes or endpoints, providing additional functionality and processing capabilities. In Node.js, middleware functions can intercept and modify the incoming request or outgoing response objects, perform additional processing, and control the flow of the request/response cycle.
Middleware functions can be used for various purposes, such as logging, authentication, authorization, error handling, request parsing, and more. Express, a popular Node.js web framework, utilizes middleware extensively.
Example: Implementing a simple logging middleware in Express.
app.use((req, res, next) => {
console.log(`[${new Date().toLocaleString()}] ${req.method} ${req.url}`);
next(); // Call next() to proceed to the next middleware or route handler
});
In this example, the middleware logs the request method and URL before passing control to the next middleware or route handler.
Q: Please explain the workflow of a reset password functionality in a Node.js application.
A: The reset password workflow typically involves the following steps:
- Request Reset: The user initiates the password reset process by requesting a password reset link or providing their email address.
- Generate Token: The server generates a unique token associated with the user and stores it in the database along with an expiration timestamp.
- Send Email: The server sends an email to the user containing the password reset link with the generated token.
- Reset Password Page: The user clicks the password reset link, which directs them to a reset password page in the application.
- Verify Token: The server verifies the token from the URL with the stored token in the database and checks if it's valid and not expired.
- Reset Password: If the token is valid, the user can enter a new password on the reset password page, and the server updates the password in the database.
- Password Updated: Once the password is successfully updated, the user receives a confirmation and can log in with the new password.
The implementation details may vary depending on the specific application and frameworks/libraries used.
Q: How do you manage asynchronous calls in Node.js to ensure they execute in a synchronized manner?
A: In Node.js, managing asynchronous calls can be done using techniques like callbacks, promises, or async/await. Here's an example of managing asynchronous calls using promises:
function asyncOperation() {
return new Promise((resolve, reject) => {
// Asynchronous operation
setTimeout(() => {
resolve('Operation completed.');
// or reject(new Error('Operation failed.'));
}, 1000);
});
}
async function executeAsyncOperations() {
try {
const result1 = await asyncOperation();
console.log(result1);
const result2 = await asyncOperation();
console.log(result2);
// ...
} catch (error) {
console.error(error);
}
}
executeAsyncOperations();
In this example, the asyncOperation
function returns a promise. By using await
, the execution of subsequent lines is paused until the promise resolves or rejects, allowing for synchronous-like code flow.
Q: Have you implemented a payment gateway in Node.js?
Q: If you're using a commenting system and need to store a large amount of data for comments and replies, how would you design the database schema?
A: When dealing with a large amount of data for comments and replies, it's essential to design a scalable and efficient database schema. Here's a possible approach:
- Comments Table: Create a table to store the comments with columns like comment_id, user_id, content, timestamp, etc.
- Replies Table: Create a separate table to store the replies with columns like reply_id, comment_id, user_id, content, timestamp, etc. Include a foreign key referencing the comment_id from the comments table.
- Indexing: Apply appropriate indexes on columns frequently used for querying, such as comment_id, user_id, or timestamp, to improve query performance.
- Pagination: Implement pagination techniques (e.g., using LIMIT and OFFSET clauses) to retrieve comments and replies in smaller chunks for better performance.
- Denormalization: Consider denormalizing the schema by including relevant information (e.g., user details) directly in the comments and replies tables to minimize joins and improve query performance.
- Database Optimization: Configure database settings, optimize query execution plans, and consider using caching mechanisms to improve overall performance.
The exact schema design and optimization strategies may vary depending on specific requirements, anticipated query patterns, and the database system being used.
Q: What is the difference between authentication and authorization?
A: Authentication and authorization are related concepts but serve different purposes:
- Authentication: Authentication is the process of verifying the identity of a user or entity. It confirms that the user is who they claim to be. Common authentication mechanisms include username/password authentication, token-based authentication, or social login (OAuth).
- Authorization: Authorization, on the other hand, is the process of granting or denying access rights to resources or actions based on the authenticated user's permissions. It determines what a user is allowed to do or access within an application. Authorization is typically based on roles, permissions, or access control rules defined by the application.
To summarize, authentication establishes the identity of a user, while authorization determines what actions or resources that authenticated user is allowed to access.
Q: What is rate limiting, and what are the best practices for implementing rate limiting when using third-party APIs? What proxy settings do you need to configure?
A: Rate limiting is a technique used to control the number of requests made to an API within a specific time frame. It helps protect the API from abuse, ensures fair usage, and prevents performance degradation. When using third-party APIs, it's important to implement rate limiting to comply with their usage policies and avoid service disruptions.
Here are some best practices for implementing rate limiting with third-party APIs:
- Understand API Rate Limits: Read and understand the rate limit policies provided by the API provider. They typically specify the maximum number of requests allowed per minute, hour, or day for each API key or user account.
- Set Appropriate Rate Limits: Determine the rate limits suitable for your application based on the API provider's guidelines and your expected usage patterns. Avoid making excessive requests that could result in rate limit violations.
- Track Request Rates: Implement mechanisms to track the number of requests made to the API within a specific time window. This can be done using counters or timers.
- Handle Rate Limit Exceedances: When the rate limit is exceeded, handle the error response returned by the API. Depending on the API provider, the error response may include headers or status codes indicating the rate limit violation. Implement appropriate actions such as delaying subsequent requests or notifying the user.
- Backoff and Retry: If a rate limit is exceeded, implement a backoff and retry mechanism to avoid overwhelming the API. Gradually increase the delay between retries to reduce the load on the API.
- Caching and Batching: Implement caching mechanisms to store API responses and serve subsequent requests from the cache when appropriate. Batch multiple API requests together to minimize the number of individual requests.
Q: What are the good ways to load a large set of images in an application?
A: Loading a large set of images in an application can pose challenges in terms of performance and user experience. Here are some good practices to consider:
- Optimize Image Sizes: Optimize the size of images by compressing and resizing them appropriately. Tools like ImageMagick, GraphicsMagick, or libraries like sharp.js can help in resizing and compressing images on the server-side. Additionally, client-side techniques like lazy loading or responsive image techniques (e.g., srcset and sizes attributes) can be used to load the appropriate image size based on the device or viewport.
- Lazy Loading: Implement lazy loading to load images only when they are about to enter the user's viewport. This technique improves initial page load performance by deferring the loading of offscreen images. Libraries like LazyLoad, Intersection Observer API, or native lazy loading attribute (with appropriate browser support) can be used for lazy loading.
- Progressive Loading: Consider using progressive loading techniques where images are loaded gradually, starting with a low-resolution or blurred version and then progressively loading higher-quality versions. This approach provides a better user experience, as users can see and interact with the images while they are loading.
- Pagination or Infinite Scrolling: If you have a large set of images, consider implementing pagination or infinite scrolling to load images in smaller batches. This approach helps avoid loading all images at once, reducing the initial load time and improving performance.
- CDN (Content Delivery Network): Utilize a CDN to deliver images efficiently. CDNs store copies of your images in multiple locations worldwide, reducing the latency and improving the loading speed for users in different regions.
- Caching: Implement caching mechanisms to store images on the client-side or on intermediate servers. This helps reduce server load and improves subsequent loading times for repeated requests.
- Optimize Network Requests: Minimize the number of network requests required to load images by combining multiple images into sprites or using image formats that support multiple images within a single file, such as WebP or SVG sprites.
By applying these practices, you can optimize the loading of a large set of images and provide a smooth and efficient user experience in your application.
When it comes to proxy settings, it depends on the specific requirements and architecture of your application. If your application is deployed behind a proxy server or load balancer, you may need to configure the proxy to handle rate limiting. This configuration can involve setting up request quotas, defining rate limit rules, or integrating a dedicated rate limiting service like Nginx or HAProxy.
Consult the documentation of your chosen proxy server or load balancer to understand the specific steps required for rate limiting configuration in your environment.
Q: Have you used web sockets before, and how do you establish a connection to a web socket?
Q: Are web sockets peer-based or server-based?
A: Web sockets are server-based. They establish a persistent, full-duplex communication channel between the client (web browser) and the server. The server-side component plays a crucial role in managing and handling web socket connections.
Once a web socket connection is established, both the client and server can send and receive messages freely, in real-time, without the need for traditional request-response cycles. This enables bidirectional communication between the client and the server, allowing them to exchange data or notifications instantly.
Web sockets provide a reliable and efficient means of real-time communication over HTTP and can be used for various applications such as chat systems, collaborative editing, live data streaming, and more.
It's important to note that while web sockets are server-based, the client-side JavaScript code plays an active role in initiating the connection, sending and receiving messages, and responding to web socket events.