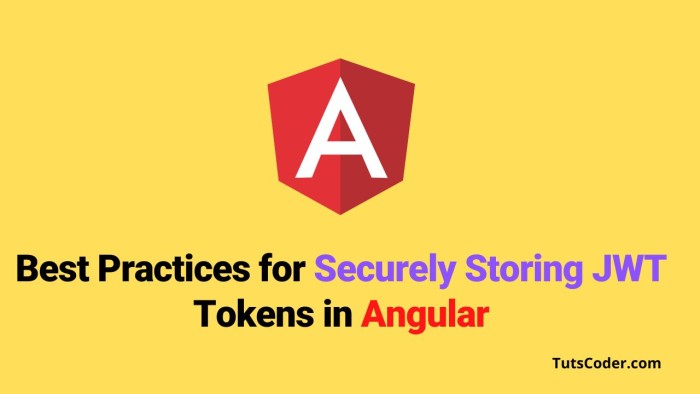
Best Practices for Securely Storing JWT Tokens in Angular Applications
JSON Web Tokens (JWTs) have become a popular method for authentication and authorization in web applications.
JWTs are stateless and easy to use, but they can also pose a security risk if not implemented properly.
In this article, we'll discuss best practices for securely storing JWT tokens in Angular applications.
What is a JWT Token?
A JWT token is a JSON object that is used to securely transmit information between parties.
JWT tokens are typically used for authentication and authorization in web applications. A JWT token consists of three parts: a header, a payload, and a signature.
The header contains metadata about the token, the payload contains the information to be transmitted, and the signature is used to verify the authenticity of the token.
Why is Secure Storage of JWT Tokens Important?
JWT tokens are used to authenticate and authorize users in web applications. If a token falls into the wrong hands, it can be used to impersonate a user and gain access to their sensitive data. For this reason, it's important to securely store JWT tokens to prevent unauthorized access.
Best Practices for Securely Storing JWT Tokens in Angular Applications
Use HTTPS
It's important to use HTTPS to encrypt the data transmitted between the client and server. HTTPS encrypts the data in transit, which makes it difficult for an attacker to intercept and read the data. Without HTTPS, an attacker could easily intercept the JWT token and gain access to the user's sensitive data.
Store JWT Tokens in Memory
One way to securely store JWT tokens is to store them in memory. When a user logs in, the server sends a JWT token to the client, which is stored in memory. The token is then sent back to the server with every request. Storing the token in memory is secure because it's not stored on the client's device.
Use Secure Cookies
Another way to store JWT tokens is to use secure cookies. Cookies are small files that are stored on the client's device. Cookies can be set to expire after a certain amount of time, which makes them a secure way to store JWT tokens. It's important to set the secure flag on the cookie to prevent it from being accessed over an unencrypted connection.
Use Local Storage or Session Storage with Caution
Local storage and session storage are two options for storing data on the client's device. However, it's important to use these options with caution because they can be vulnerable to cross-site scripting (XSS) attacks. If an attacker can inject malicious code into the application, they could potentially access the JWT token stored in local storage or session storage.
Example: Storing JWT Tokens in Memory
To store JWT tokens in memory, you can use an Angular service to manage the token. Here's an example service that stores the token in memory:
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class AuthService {
private token: string;
constructor() { }
public setToken(token: string): void {
this.token = token;
}
public getToken(): string {
return this.token;
}
}
Conclusion
JWT tokens are a popular method for authentication and authorization in web applications, but they can pose a security risk if not implemented properly.
By following these best practices for securely storing JWT tokens in Angular applications, you can help prevent unauthorized access to your users' sensitive data.
Using HTTPS, storing JWT tokens in memory or secure cookies, and using local storage or session storage with caution are all ways to help keep your users' information safe. Remember to always prioritize security when implementing JWT tokens in your application..